The blog post introduces a concept of currying, which is an important concept in functional programming including Haskell.
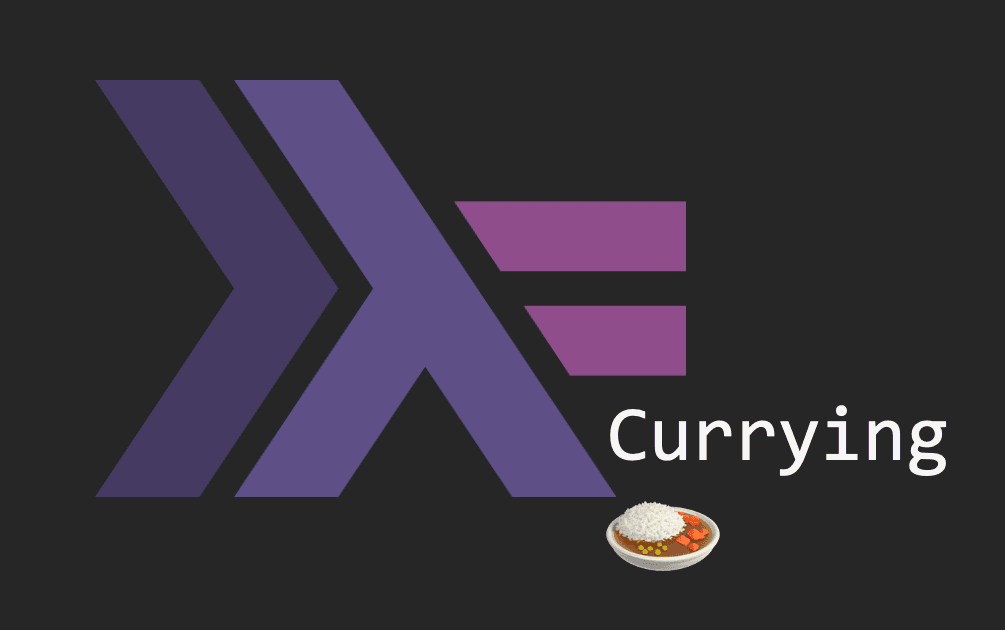
Currying
Currying is one of the concepts in functional programming that many beginners get confused about. Cliché, but currying is not about eating curry; it is about a function with multiple arguments being interpreted as nested functions with one input each.
func:: a -> b -> c -> d
func:: a -> (b -> (c -> d))
This concept allows Haskell to behave unexpectedly to some programmers. Let’s look at a simple example.
add:: Int -> Int -> Int
add x y = x + y
The above function add
takes x
and y
as inputs. However, we can refactor the function to the following:
add :: Int -> Int -> Int
add x = (\y -> x + y)
Here, the function takes x
as the only input, but y
is in the function.
In imperative programming languages, this should be unacceptable.
However, in Haskell, it is legal because a function is just a value in Haskell.
A function returning a function is totally okay. In the case of the add function,
the above is returning another function that adds x
to the y
provided next.
add 1 --- Output: (\y -> 1 + y)
add 1 2 --- Output: 3
When we transform a function into a function with fewer parameters, we call it partial function application, and it is widely used in functional programming.
Real-Life Example
Let's see how partial function application is used in a real-life context. The map
function we covered in the previous article is a great example.
If you remember correctly, you know that the map
function can be applied as follows:
map (\x -> x + 2) [1,2,3,4,5] --- Output: [3,4,5,6,7]
However, what if we want to use the same anonymous function multiple times? Do we need to type (\x -> x + 2)
over and over again?
Luckily, we can use partial function application here.
add2ToList = map (\x -> x + 2)
Boom! We created a new function that can add 2 to the elements of a list. The function can be reused multiple times.
Exercises
This is an exercise section where you can test your understanding of the material introduced in the article. I highly recommend solving these questions by yourself after reading the main part of the article. You can click on each question to see its answer.
Resources
- Philipp, Hagenlocher. 2020. Haskell for Imperative Programmers #7 - Partial Function Applications and Currying. YouTube.