The blog post introduces higher-order functions and anonymous functions, which are all important concepts in Haskell.
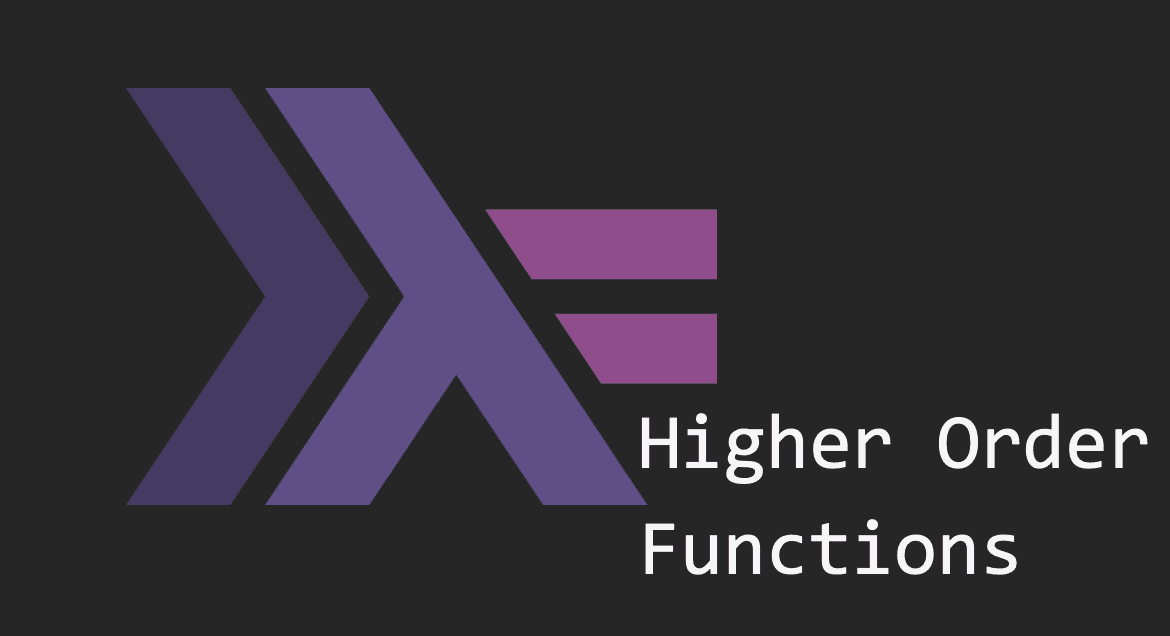
Higher Order Functions
As I have briefly mentioned before, functions are treated just like values in Haskell. This means that functions can be passed as parameters to another higher-order function.
app:: (a -> b) -> a -> b
app f x = f x
The above function app
takes a function f
and a value x
as inputs, and run the function f
on x
.
This means that the following can happen in Haskell.
addOne x = x + 1
app addOne 3 --- Output 4
This might not look like a useful feature but it will come in handy.
Anonymous Functions
As you look at various examples like addOne
that just perform simple operation,
you might be thinking if you have to come up with a name for every single function and define
it properly. Luckly, we have anonymous functions in Haskell which can simplify the syntax
like the following.
(\<args> -> <expr>)
--- <args>: arguments/inputs of an anonymous function
--- <expr>: expressions/operations of an anonymous function
--- Example
(\ x y z -> x + y + z) 1 2 3 --- Output: 6
By using anonymous functions, we can build two of the most useful higher-order functions in Haskell.
Map, Filter
The following functions, map
and filter
, are so useful, and you should understand and
remember how they work.
--- Map (perform operation on every element in a list)
map::(a -> b) -> [a] -> [b]
map (\x -> x + 1) [1,2,3,4,5] --- Output: [2,3,4,5,6]
--- Filter (remove elements that do not meet the condition(s))
filter::(a->Bool) -> [a] -> [a]
filter (\x -> x > 2) [1,2,3,4,5] --- Output: [3,4,5]
Exercises
This is an exercise section where you can test your understanding of the material introduced in the article. I highly recommend solving these questions by yourself after reading the main part of the article. You can click on each question to see its answer.
Resources
- Philipp, Hagenlocher. 2020. Haskell for Imperative Programmers #6 - Higher Order Functions and Anonymous Functions. YouTube.