The blog post introduces the concept of templates in C++.
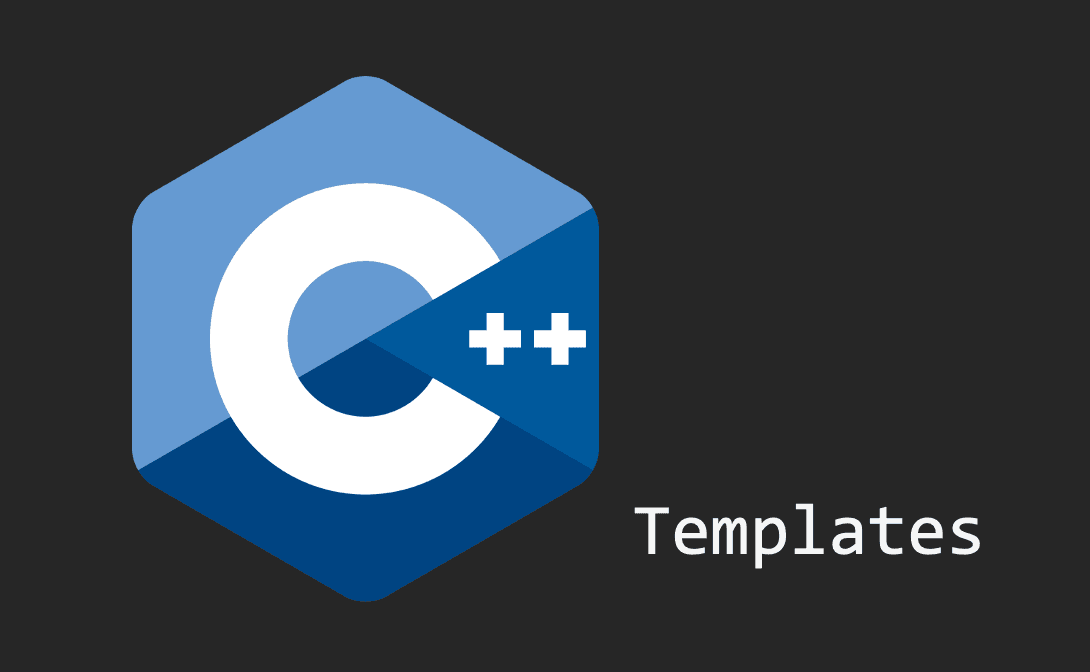
Function Templates
Since both C and C++ are compiled languages, the types of inputs and outputs need to be specified for every function. However, this can lead to duplication, as shown below:
int sum (int a, int b) {
return a + b;
};
double sum (double a, double b) {
return a + b;
};
The logic of both functions is identical, but we had to define them separately—until now. In C++, we can use function templates to create a type variable and define both functions in one go:
template <typename T>
T sum (T a, T b) {
return a + b;
};
int main () {
cout << sum<int>(3, 4) << endl;
cout << sum(3.1, 4.4) << endl; // compiler can infer the type
return 0;
}
The compiler will recognize which type to use and automatically generate template functions for us. This reduces duplication and makes refactoring easier.
Class Templates
You can also create class templates, which allow us to define flexible classes, as shown below:
template <typename T>
class Array {
public:
T array[10];
void fill (T value) {
for (int i; i < 10; i++) {
array[i] = value;
}
};
};
This allows us to create arrays for strings, integers, doubles, and so on. You can also create multiple type variables and template variables with templates:
template <typename T, int length>
class Array {
public:
T array[length];
void fill (T value) {
for (int i; i < length; i++) {
array[i] = value;
}
};
};
int main () {
Array<int, 8> arr;
return 0;
}
The compiler will generate template classes using the specified type or value of the template variable from the class templates.
Caution
While templates bring immense flexibility and reduce duplication, there are some common mistakes beginners tend to make. Firstly, remember that template functions and classes are generated at compile time, not at runtime. This means the following is not allowed:
int main () {
int x;
cin >> x;
Array<int, x> arr; // Err!
return 0;
}
The cin
function takes input from the console at runtime. Therefore, the above code fails to compile.
Another common mistake occurs when overloading functions with function templates:
template <typename T>
T sum (T a, T b) {
return a + b;
};
T sum (T a, T b, T c) {
return a + b + c;
};
int sum (int a, int b) {
return a + b;
};
The above function overloading works because there is no ambiguity. When three inputs are provided, the second function is used. When two integers are provided, the last function is called, and when two non-integer inputs are given, the first template function is utilized. However, the following example introduces ambiguity:
template <typename T>
T sum (T a, int b) {
return a + b;
};
T sum (int a, T b) {
return a + b;
};
When one of the inputs is an integer, the other input and the output must also be integers. The compiler uses this to decide which template to choose, but it cannot decide between the two functions in this case, causing ambiguity. Always check for potential ambiguity when overloading functions with function templates.
Exercises
From this article, there will be an exercise section where you can test your understanding of the material introduced in the article. I highly recommend solving these questions by yourself after reading the main part of the article. You can click on each question to see its answer.
Resources
- CodeAesthetic. 2023. The Flaws of Inheritance. YouTube.
- Portfolio Courses. 2022. Abstract Classes And Pure Virtual Functions | C++ Tutorial. YouTube.